How to Create Your First Next.js Serverless App
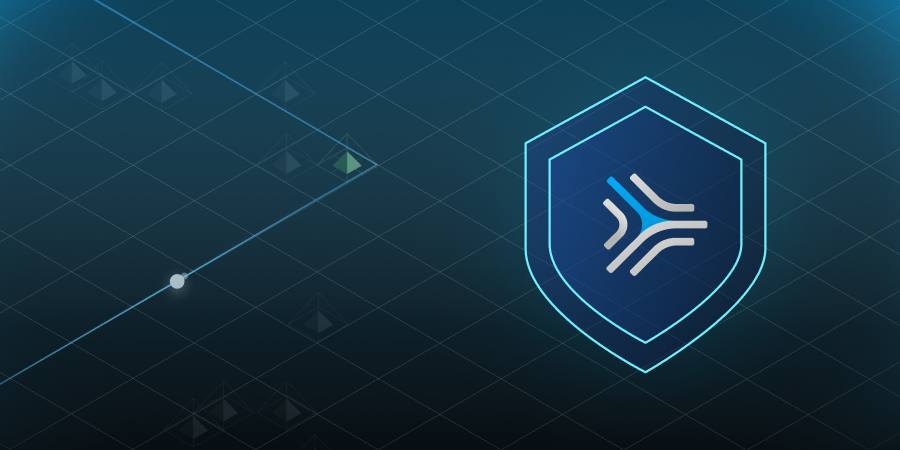

Serverless architecture is a relatively new trend in the cloud computing era. It helps IT organizations provide business customers with only the necessary and required resources to run applications -- like a Next.js app -- when and where they're needed.
This post will walk you through how to create a serverless Next.js app from scratch. You'll learn how to take advantage of the combination of Next.js and MongoDB and deploy it on Vercel to create a simple web app that can handle millions of requests.
What Is Serverless?
Serverless is a way of building applications in which the developer only has to worry about their application code. At the same time, the server and its infrastructure are automatically handled by a third-party service.
Serverless computing is a way to deploy applications where the cloud provider is responsible for running the applications and scaling them automatically. Serverless development allows for an event-driven computing environment and uses a pay-per-use model. It's a relatively new technology, but it's a natural fit for many applications and dramatically simplifies the development life cycle.
5 Benefits of Serverless Applications
Serverless architecture has significantly impacted how developers build and manage applications. The following are some of the benefits that serverless offers:
- Pay for what you use—Whether you need a lot of computing power to build your application or just a little to scale it, you only need to pay for what you use.
- No servers to manage—Developers no longer have to worry about configuring and managing servers.
- Secure by design—Serverless computing is a cloud-based solution and, therefore, encryption and security are built in.
- Reusable code—Serverless computing uses functions developers can reuse across different projects.
- Scalable and flexible—The ability to scale serverless applications quickly and efficiently makes them ideal for dynamic applications.
Now that we understand the benefits of serverless, let’s start developing a very minimal to-do application using Next.js.
Practical Guide to Serverless Application
Let’s start making our very first serverless application using Next.js. We’ll be using Tailwind CSS and HyperDev UI for pre-built components. Before getting into Next.js, let's first have a sneak peek into what Next.js is.
Next.js is one of the most popular frameworks in the web development community. It's built on top of React.js. This framework provides developers with a lot of features, such as SSR (server side rendering), SFS (static file serving), hot-reloading, and much more.
To speed up our process, I've created a boilerplate application. First, clone the repository and start the development server.
To start the application, follow the below-mentioned steps:
1. Clone the repository using the command
git clone https://github.com/theinfosecguy/hs-serverless
2. Change the directory using the command
cd hs-serverless
3. Start the development server using the command
npm run dev
Now that we've started our application, it’s time to make changes and understand how things work. The first step would be setting up MongoDB Atlas to save our data.
Step 1: Set Up MongoDB Atlas
First, we’ll have to set up a free MongoDB cluster and use the MongoDB Connect URL in our application’s .env file to connect to our database.
To create a free MongoDB Cluster, go to the registration page and create your account. After successful registration, you’ll get the following screen. Choose the Shared environment and click Create.
Then, choose any cloud service provider and region based on your choice and click Create Cluster. While the cluster is being created, MongoDB will ask security questions for the cluster.
Image 1: MongoDB plan screen
Next, enter your desired username and password. Make sure the password is secure. Then, click on Create User after entering a username and password to add a user. Now, let’s move to the next question.
Image 2: Choose username and password
Choose the IP address from where you want to connect to the database. You can click on the Add My IP Address button to add your IP address.
Once done, click on Finish and Close to complete the setup.
Image 3: IP range for DB connection
To connect the cluster, follow the below-mentioned steps:
- Go to the Database tab from the sidebar.
- Click on Cluster Name (Cluster 0 if you didn’t name it).
- Once done, click on the Connect button, which will open a pop-up menu.
- Click on Connect your application and copy the driver code.
Step 2: Connect Next.js and MongoDB
Now that we have the MongoDB Driver URL, go to the .env file and paste the URL after MONGO_URL=. After entering the URL, the server will automatically restart once you save the .env file.
This is all you need to do to set up a serverless application. But wait—where are the serverless functions?
Step 3: Understanding a Serverless Application
We've successfully set up the application. Therefore, the directory structure of the application should look like this:
Image 4: Directory structure
Here, you can see we have a folder named pages, which contains another folder named api including our serverless functions. Let’s explore these functions.
If you've tried to explore the application by opening http://localhost:3000 in your browser, it'll open the homepage.
NOTE: Any file in the pages directory will automatically create a route with the name of the file (including the api directory).
For example, we have todo.js, which makes a route/todo in our application.
We have an input form on this page that's used to add any to-do task in our database. The Add Task button here calls a serverless function that inserts data in our collection.
Now, enough theory about serverless function—let’s understand serverless functions in depth. We have two different functions, named get and save. You can see two files with the same name in the api directory.
Function 1: Get Serverless Function
We use this function to fetch to-do items from the database. In this case, we're using MongoClient from the mongodb package to connect to our database. After successful connection, we use the find function to find all to-do items from our collection named to-do, which will return all the items from our database.
Function 2: Save Serverless Function
We use this function to save to-do items from the database. In this case, we'll use the same MongoClient from the mongodb package to connect to our database. After successful connection, we use the insertOne function to insert data in our collection.
Using Serverless Functions
We're using both the serverless functions on the /todo route. Whenever a user clicks on the Add Task button, the action calls the save function.
Separately, we use the get function in useEffect to fetch the tasks every time a user lands on the page.
We can use fetch or Axios to hit the API route to use these functions.
Step 4: Deployment Using Vercel
Now that we've successfully developed a basic serverless application, it’s time to deploy it on Vercel, which founded Next.js. To deploy the application, follow the below-mentioned steps:
- Upload your application to GitHub.
- Go to Vercel Login and log in using GitHub.
- Click on New Project, choose your repository, and click Import.
- Use the default settings and start deployment.
Once the deployment is complete, you can use your application by visiting the deployment link provided by Vercel.
NOTE: This is a dummy application for tutorial purposes only and might not be secure enough. Check out our free API Security Guide to learn about API security.
Conclusion
Serverless architecture, a new trend in the IT industry, is a method of building applications that allows you to focus more on the development process and less on managing servers.
This blog has covered the basic steps for developing your first serverless application using Next.js with a focus on what serverless functions are and how they work.
So, what are you waiting for? Start building your own serverless app right now. But don't forget to secure them. To know how to secure and enhance your API security for cloud-native apps, feel free to visit our website.
This post was written by Keshav Malik. Keshav is a full-time developer who loves to build and break stuff. He is constantly on the lookout for new and interesting technologies and enjoys working with a diverse set of technologies in his spare time. He loves music and plays badminton whenever the opportunity presents itself.
The Inside Trace
Subscribe for expert insights on application security.